前言
去年暑假学了一下vue
,这个假期想学一下react
、typescript
(可能要鸽)
基础
更新已渲染元素
1 2 3 4 5 6 7 8 9 10 11 12
| function tick() { const element = ( <div> <h1>Hello,world</h1> <h2>It is{new Date().toLocaleTimeString()}</h2> </div> ) ReactDOM.render(element, document.getElementById('root')); }
setInterval(tick,100)
|
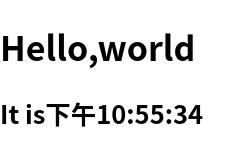
计时器
setInterval 每几秒执行一次
setTimeout 几秒后再执行
组件定义
1 2 3 4 5 6 7 8 9 10 11
| class Welcome extends React.Component { render() { return <h1>Hello, {this.props.name}</h1>; } }
function Welcome(props) { return <h1>Hello, {props.name}</h1>; }
|
组件渲染
App.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| function Welcome(props) { return <h1>Hello, {props.name}</h1>; }
function App() { return ( <div> <Welcome name="Sara" /> <Welcome name="Cahal" /> <Welcome name="Edite" /> </div> ); } export default App;
|
index.js
1 2 3 4
| import App from './App' ReactDOM.render( <App />, document.getElementById('root'));
|
效果
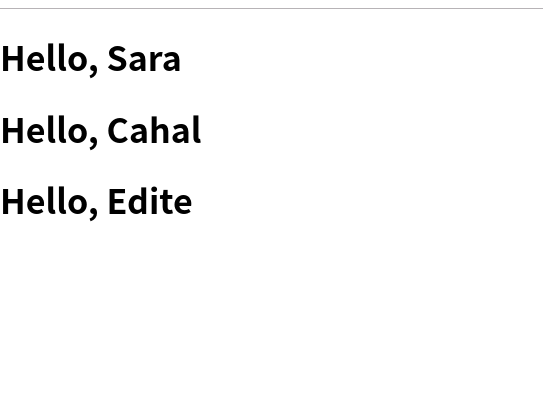
组件名首字母要大写
1 2 3 4 5 6 7 8
| function Sum(props) { return (<div>{props.a+props.b}</div>); } ReactDOM.render( <Sum a={1}b={2}/>, document.getElementById('root'));
|
整型必须加 {}
,字符串可以直接加双引号
事件
1 2 3
| const refresh = (src) => event => { }
|
需要 => event=>
如果缺少会直接执行,当做执行后的结果赋值给变量
路由(react-router-dom)
基础
1 2 3 4 5 6 7 8 9
| return ( <React.Fragment> <HashRouter> <Switch> <Route path={"/home"} component={Home} /> </Switch> </HashRouter> </React.Fragment> )
|
路由重定向
1 2 3 4 5 6 7 8 9 10 11
| return ( <React.Fragment> <HashRouter> <Switch> <Route exact path="/" render={() => <Redirect to='/home'></Redirect>}></Route> <Route path={"/home"} component={Home} /> </Switch> </HashRouter> </React.Fragment> )
|
访问根目录时会跳转到/home
路由跳转(withRouter)
参考https://github.com/brickspert/blog/issues/3
组件放在标签内使用 withRouter
来做
1 2 3 4 5 6 7 8 9
| function Router(){ return ( <HashRouter> <Switch> <Route path="/" component={Base} /> <Route path="/login" component={Login} /> </Switch> </HashRouter> )}
|
然后设置方法
1 2 3 4 5 6 7 8 9 10
| function Base(props){ const toLoginRoute = () => { props.history.push("/login") } return ( <Button onclick={toLoginRoute}></Button> ) }
export default withRouter(Base);
|